As I said in parts 1 & 2, please ignore this, it’s dumb.
Did you know?: There are different infinities? There are bigger ones and smaller ones, countable and uncountable ones and more!? If you think about your positive natural numbers1 you have 1, 2, 3, 4…etc. You can “count” every number to infinity, this is a Countable Infinity2. Think about all the decimals between 0 and 1: 0.1, 0.001, 0.0001…0.2, 0.002…etc. There is no “start” there is no “first decimal after zero,” there is always a smaller number, this is an Uncountable Infinity3.
A laser4 is a device that emits a single wavelength of light. So lasers can generate that “pure color” they can make a light that is 600nm pure-yellow and only 600nm pure-yellow. Theoretically we could create a rainbow using lasers comprised of all of the colors….Roy G. Biv…But what if we want more colors than just the base 7? I might want a little blue-green in my rainbow. We can try to make more lasers to fill in the gaps, but soon we see we are dealing with an uncountable infinity. Given any two colors, no matter how close they are, there is always a color that exists between the two of them.
Light is both a particle and a wave: You have heard that, right? There are multiple ways to understand this, and if you have made it this far, you now have one.
Particle: A piece of light (a photon) such as that a laser emits, or anything because we are talking about one particle, is a single color (and as you now understand, can never be purple) this is the light particle.
Wave: The wavelength of light can be any number in the uncountable infinity between 400nm and 665nm. It could be 400nm, 400.01nm, 400.0000001nm, 432.0001nm, 634.5445644nm, anything. This is the light wave.
When we see light (even when you look at a laser, ambient light and such) we are seeing the combination of the wave and the particle. A vast array of Individual particles that each have a specific color that is somewhere in our visible spectrum. Some are red, some are aquamarine, some are blue-green, some are #AAEEFF.
Do you think any two pieces of light are ever exactly the same? If you were to measure the wavelength of any and all of them out to an infinite amount of decimals, would some of them be the same, or if you keep looking do they all eventually differ?
Why are people so keen to call something purple but not blue-green? Purple is great, it gives me a good idea of what something looks like…But if you are going to give me purple, why not elevate all colors to the same level? Orange? Was it red-orange or yellow-orange. Do we just not have the language? Ok, roarnge and yorange, and you put the emphasis where the color puts it – “ROarnge” is a very redy-orange, “roarNGE” is a very orangy-red. I LOVE colors! Tell me about what you really see!
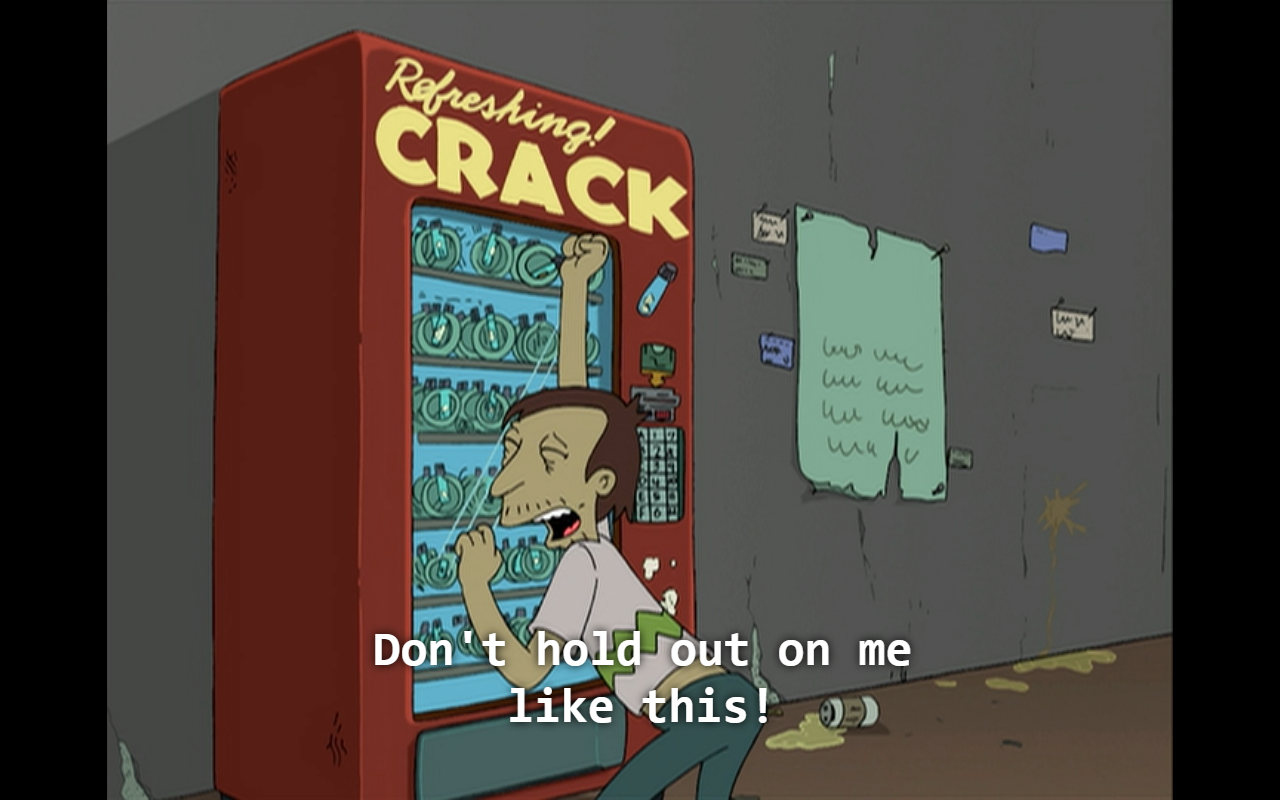
1 https://en.wikipedia.org/wiki/Natural_number
2 http://mathworld.wolfram.com/CountablyInfinite.html
3 http://mathworld.wolfram.com/UncountablyInfinite.html
4 https://en.wikipedia.org/wiki/Laser
Part 1: http://www.mattevanoff.com/2024/06/purple-part-one/
Part 3: http://www.mattevanoff.com/2024/06/purple-part-three/